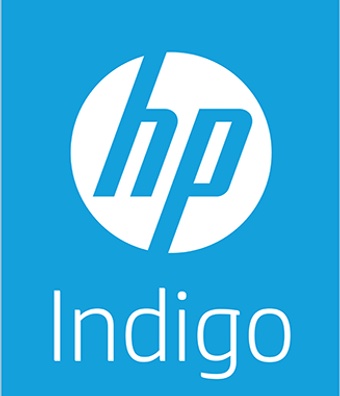
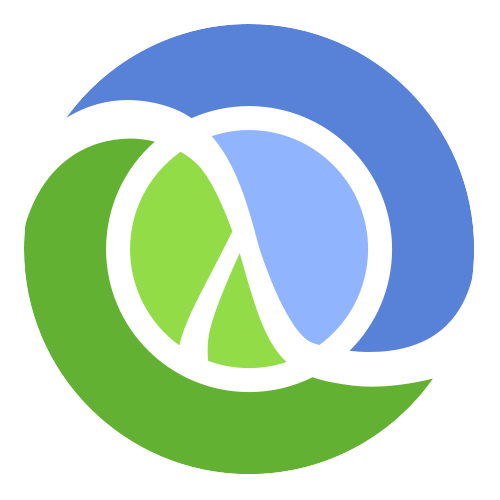
Leverage homoiconicity and immutability to empower your developing experience |
(defn foo [a b]
(let [x (/ a b)
y (+ a b)]
(+ x y)))
(foo 2 3)
(defn foo [a b]
(let [x (/ a b)
y (+ a b)]
(println "x: " x)
(println "y: " y)
(+ x y)))
(foo 2 3)
dbg
macro(defn foo [a b]
(+ (dbg (* a b))
(/ (dbg b) 3)))
(foo 12 2)
☕ How is it related to homoiconicity?
(defmacro dbg[x]
(when *assert*
`(let [x# ~x]
(println (str '~x ": ") x#)
x#)))
def
like crazy(defn foo [a b]
(let [x (/ a b)
y (+ a b)]
(def x x)
(def y y)
(+ x y)))
(foo 2 3)
(+ x y)
☕ How is it related to immutability?
scope-capture
Demo on the IDE
Use the dbg
macro
Add sc.api
to your profile.clj
Play with sc.api