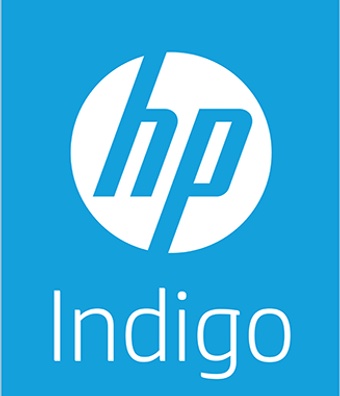
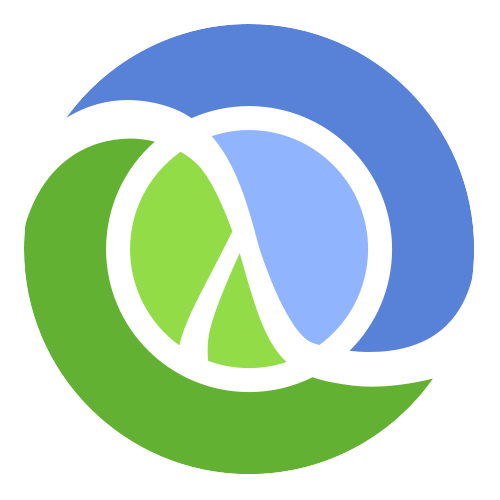
An elegant syntactic sugar
Destructuring the first 2 values
| Skip some items with
|
Split a vector into
| Keep access to the whole sequence
|
Choose local names and provide keys
(let [{the-x :x the-y :y} {:x 5 :y 7}]
(+ the-x the-y))
Most useful piece of destructuring
(let [{:keys [x y]} {:x 5 :y 7}]
(+ x y))
Keep access to the whole map
(let [{:keys [x y] :as p} {:x 5 :y 7}]
p)
Can you guess how to destructure a map that is inside a vector?
For example
[{:a 1 :b 2}]
?
Solution
(let [[{:keys [a b]}] [{:a 1 :b 2}]]
a)
Write a function
foo
that receives a map with 3 keys:a
,:b
and:c
and returns the greatest value in the map, where:
:a
and:b
are required
:c
is optional with a default value of 10
(defn foo [])