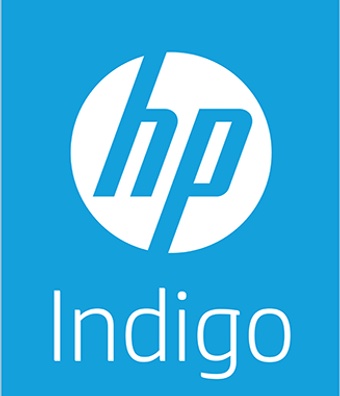
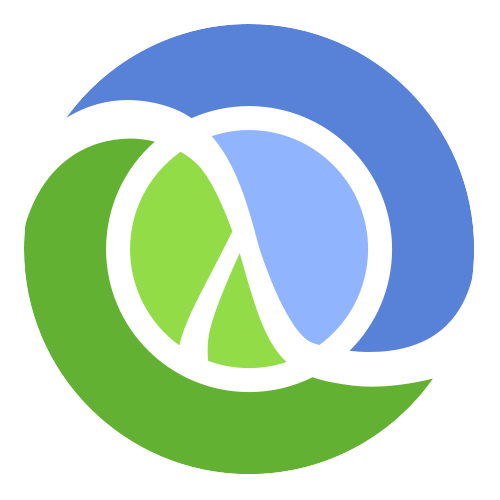
The syntax of the code is the same as the syntax of the data. |
|
|
A function is an entity that receives multiple things and return a thing.
In functional programming the things that functions receive and return are allowed to be functions.
We say that functions are first class citizens. |
We create a function with defn
(defn bar
"bar is a function with documentation"
[a b c]
b)
We call a function like this
(bar 1 2 3)
Live demo
Instead of representing data with classes, we use maps.
The maps are immutable.
(def m {"a" 1})
(assoc m "b" 2)
m
Klipse: Clojure REPL online
ClojureDocs: Documentation with Examples
Clojurians: Slack Channel
Cursive: Clojure IDE based on IntelliJ
Register to Clojurians: Slack Channel
Post at least 1 question on Clojurians
10 minutes of ClojureScript koans every day
Solve this exercise
Write a function hello-world that receives a name and prints "Hello <name>!" to the console.
Write a function hello-worlds that receives multiple names and prints "Hello <name>!" to the console for each name
Write tests for both functions